Introduction
Welcome to the world of Data Structures and Algorithms (DSA)! If you’re starting your programming journey or looking to strengthen your fundamental computing knowledge, you’ve come to the right place. DSA forms the backbone of computer science and is essential for writing efficient, optimized code that can handle real-world problems.
But what exactly are data structures and algorithms, and why are they so important? Data structures are specialized formats for organizing and storing data, while algorithms are step-by-step procedures for solving specific problems. Together, they form the building blocks that power everything from simple applications to complex systems like search engines, social networks, and operating systems.
Understanding Data Structures
What Are Data Structures?
Data structures are specialized ways of organizing data to perform operations efficiently. Think of them as containers designed to store data in ways that make access, modification, and manipulation more effective based on specific use cases.
Just as you wouldn’t use a spoon to cut a steak or a knife to eat soup, different data structures are optimized for different operations. Choosing the right data structure can dramatically impact your program’s performance and resource usage.
Why Is DSA Important?
- Optimized Performance: Efficient data structures and algorithms can greatly reduce the time and memory required for your applications.
- Problem Solving: Mastering DSA improves your problem-solving skills, enabling you to break down complex problems into manageable pieces.
- Interview Readiness: Many tech companies focus on DSA in their interviews. A strong grasp of these concepts can set you apart from the competition.
- Foundation for Advanced Topics: Understanding DSA is a prerequisite for exploring more advanced topics like machine learning, system design, and artificial intelligence.
Types of Data Structures
Data structures generally fall into two categories:
1. Primitive Data Structures
These are the basic data types built into programming languages:
- Integers
- Floating-point numbers
- Characters
- Boolean values
2. Non-Primitive (Abstract) Data Structures
These are more complex structures built using primitive data types:
Linear Data Structures:
- Arrays
- Linked Lists
- Stacks
- Queues
Non-Linear Data Structures:
- Trees
- Graphs
- Hash Tables
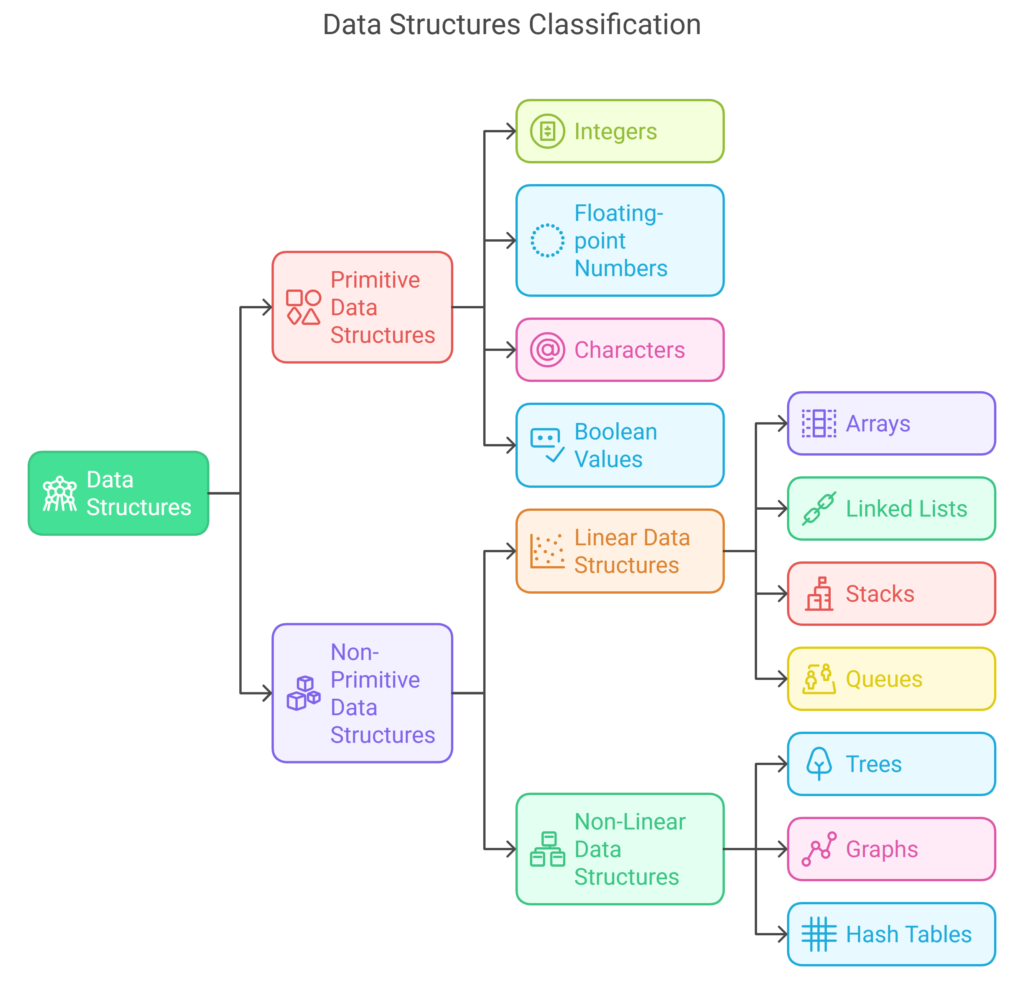
Essential Data Structures in Detail
Arrays
Arrays are the simplest and most widely used data structure, consisting of elements stored in contiguous memory locations.
// Declaring and initializing an array
int numbers[5] = {1, 2, 3, 4, 5};
// Accessing elements (zero-indexed)
std::cout << numbers[0]; // Outputs: 1
std::cout << numbers[4]; // Outputs: 5
// Modifying elements
numbers[2] = 10; // Array becomes {1, 2, 10, 4, 5}
CKey Characteristics:
- Fixed size (in static arrays)
- Constant-time access to elements (O(1))
- Elements must be of the same data type
- Memory efficiency
Common Applications:
- Storing and accessing sequential data
- Implementing other data structures
- Temporary storage for computational operations
Linked Lists
Linked lists consist of nodes where each node contains data and a reference to the next node in the sequence.
struct Node {
int data;
Node* next;
};
// Creating a simple linked list
Node* head = new Node{1, nullptr};
head->next = new Node{2, nullptr};
head->next->next = new Node{3, nullptr};
CKey Characteristics:
- Dynamic size
- Efficient insertions and deletions
- Non-contiguous memory allocation
- Linear time access to elements (O(n))
Types of Linked Lists:
- Singly Linked List (navigation in one direction)
- Doubly Linked List (navigation in both directions)
- Circular Linked List (last node points to first node)

Practice Exercise: Implement a function that reverses a singly linked list.
Stacks
Stacks follow the Last-In-First-Out (LIFO) principle, where the last element added is the first one to be removed.
#include <stack>
std::stack<int> myStack;
// Push operations
myStack.push(1);
myStack.push(2);
myStack.push(3);
// Pop operations
std::cout << myStack.top(); // Outputs: 3
myStack.pop();
std::cout << myStack.top(); // Outputs: 2
CKey Operations:
- Push: Add an element to the top
- Pop: Remove the top element
- Peek/Top: View the top element without removing it
Common Applications:
- Function call management (call stack)
- Expression evaluation
- Backtracking algorithms
- Undo mechanisms in applications
Queues
Queues follow the First-In-First-Out (FIFO) principle, where the first element added is the first one to be removed.
#include <queue>
std::queue<int> myQueue;
// Enqueue operations
myQueue.push(1);
myQueue.push(2);
myQueue.push(3);
// Dequeue operations
std::cout << myQueue.front(); // Outputs: 1
myQueue.pop();
std::cout << myQueue.front(); // Outputs: 2
CKey Operations:
- Enqueue: Add an element to the back
- Dequeue: Remove the front element
- Front: View the front element without removing it
Common Applications:
- Task scheduling
- Print queue management
- Breadth-first search
- Buffering in data transfers
Trees
Trees are hierarchical data structures with a root node and branches leading to child nodes.
Binary Trees
A binary tree is a tree where each node has at most two children.
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
};
// Creating a simple binary tree
TreeNode* root = new TreeNode{1, nullptr, nullptr};
root->left = new TreeNode{2, nullptr, nullptr};
root->right = new TreeNode{3, nullptr, nullptr};
CBinary Search Trees (BST)
A binary search tree maintains the property that the left child of a node contains a value less than the node’s value, and the right child contains a value greater than the node’s value.
Key Characteristics:
- Efficient searching, insertion, and deletion (O(log n) on average)
- Ordered structure
- Simplified lookup operations
Practice Exercise: Implement a function to check if a binary tree is a valid binary search tree.
Graphs
Graphs consist of nodes (vertices) connected by edges, representing relationships between objects.
// Adjacency list representation
std::vector<std::vector<int>> graph(5); // 5 vertices
// Add edges
graph[0].push_back(1); // Edge from vertex 0 to 1
graph[0].push_back(4);
graph[1].push_back(2);
graph[1].push_back(3);
graph[1].push_back(4);
graph[3].push_back(2);
graph[3].push_back(4);
CTypes of Graphs:
- Directed vs. Undirected
- Weighted vs. Unweighted
- Cyclic vs. Acyclic
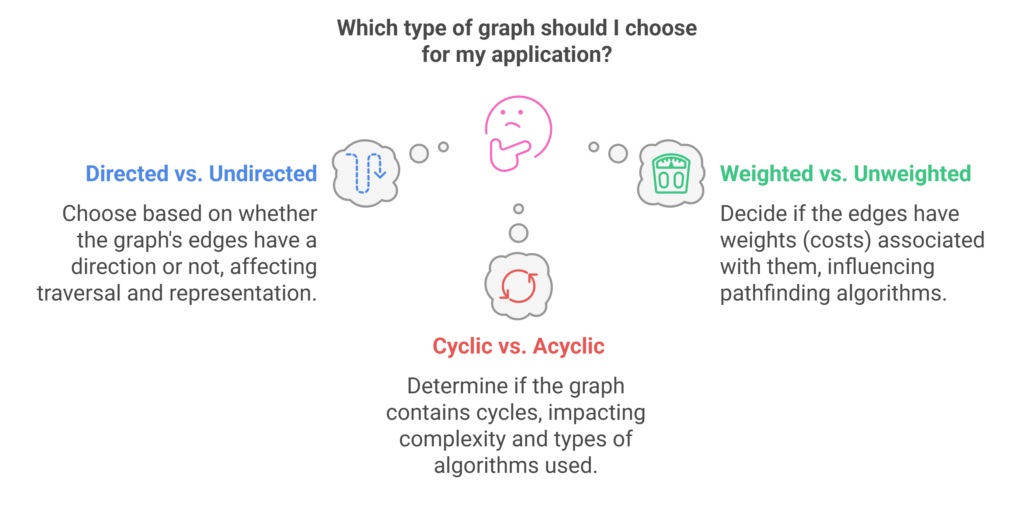
Common Applications:
- Social networks
- Road networks and maps
- Web page connections
- Recommendation systems
Hash Tables
Hash tables use a hash function to map keys to values, allowing for efficient lookup, insertion, and deletion operations.
#include <unordered_map>
std::unordered_map<std::string, int> ages;
// Inserting key-value pairs
ages["Alice"] = 25;
ages["Bob"] = 30;
ages["Charlie"] = 22;
// Accessing values
std::cout << ages["Bob"]; // Outputs: 30
// Checking if a key exists
if (ages.find("David") != ages.end()) {
std::cout << "David's age is known";
} else {
std::cout << "David's age is unknown";
}
CKey Characteristics:
- Constant-time average case operations (O(1))
- Unordered storage
- Key-value paired storage
Common Applications:
- Database indexing
- Caching
- Symbol tables in compilers
- Implementing sets and maps
Understanding Algorithms
What Are Algorithms?
Algorithms are step-by-step procedures for solving specific problems. They take input, process it through a series of well-defined operations, and produce output. A good algorithm is:
- Correct: It should solve the problem accurately
- Efficient: It should use resources (time and space) optimally
- Finite: It should terminate after a finite number of steps
- Deterministic: Given the same input, it should always produce the same output
Algorithm Analysis
Understanding the efficiency of algorithms is crucial for writing performant code. We analyze algorithms using Big O notation, which describes the worst-case time or space complexity.
Common Time Complexities
NotationNameExample
O(1)ConstantArray access, hash table lookup
O(log n)LogarithmicBinary search
O(n)LinearLinear search
O(n log n)LinearithmicEfficient sorting algorithms (merge sort, quicksort)
O(n²)QuadraticSimple sorting algorithms (bubble sort, insertion sort)
O(2ⁿ)ExponentialRecursive calculation of Fibonacci numbers
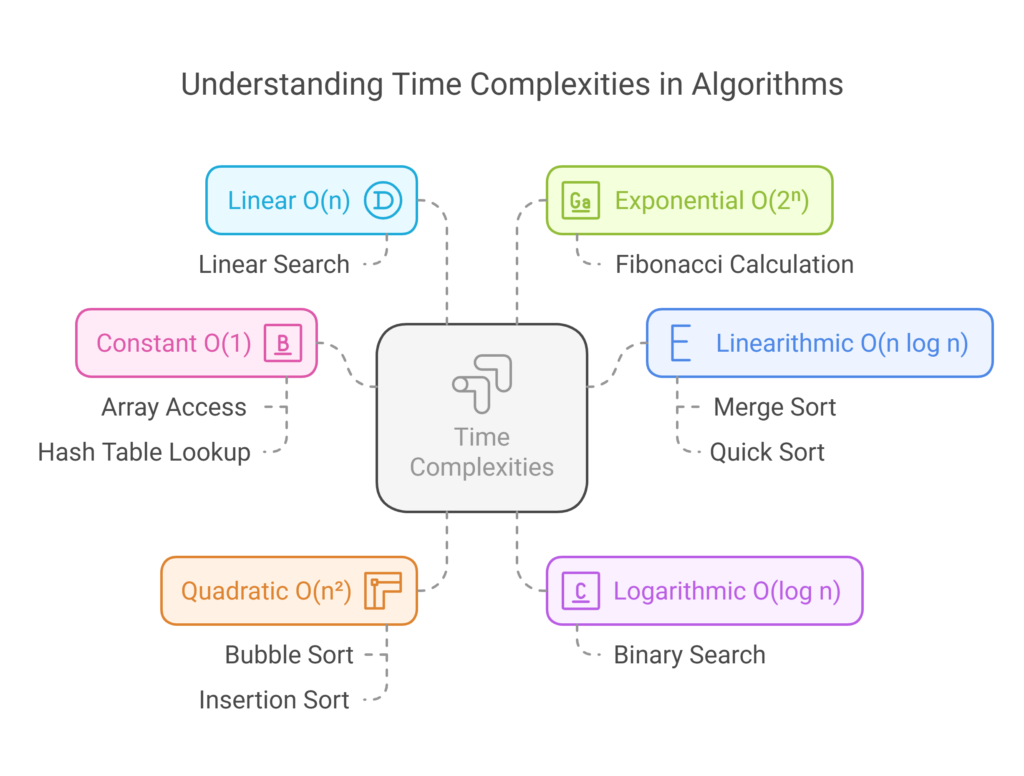
Copyright: https://www.courseflash.com
Practice Exercise: Analyze the time complexity of this function:
int sum(int arr[], int n) {
int total = 0;
for (int i = 0; i < n; i++) {
total += arr[i];
}
return total;
}
CEssential Algorithms
Searching Algorithms
Linear Search
The simplest searching algorithm that checks each element sequentially.
int linearSearch(int arr[], int n, int x) {
for (int i = 0; i < n; i++) {
if (arr[i] == x) {
return i; // Return index if found
}
}
return -1; // Return -1 if not found
}
C- Time Complexity: O(n)
Binary Search
A divide-and-conquer algorithm that works on sorted arrays by repeatedly dividing the search range in half.
int binarySearch(int arr[], int left, int right, int x) {
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == x) {
return mid; // Return index if found
}
if (arr[mid] < x) {
left = mid + 1; // Look in right half
} else {
right = mid - 1; // Look in left half
}
}
return -1; // Return -1 if not found
}
C- Time Complexity: O(log n)
Practice Exercise: Implement binary search recursively.
Sorting Algorithms
Bubble Sort
A simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order.
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
C- Time Complexity: O(n²)
Merge Sort
A divide-and-conquer algorithm that divides the input array into two halves, sorts them separately, and then merges them.
void merge(int arr[], int left, int mid, int right) {
int n1 = mid - left + 1;
int n2 = right - mid;
// Create temporary arrays
int L[n1], R[n2];
// Copy data to temp arrays
for (int i = 0; i < n1; i++)
L[i] = arr[left + i];
for (int j = 0; j < n2; j++)
R[j] = arr[mid + 1 + j];
// Merge the temp arrays back into arr[left..right]
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
// Copy remaining elements
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void mergeSort(int arr[], int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
// Sort first and second halves
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
// Merge the sorted halves
merge(arr, left, mid, right);
}
}
C- Time Complexity: O(n log n)
Quick Sort
Another divide-and-conquer algorithm that picks an element as a pivot and partitions the array around the pivot.
int partition(int arr[], int low, int high) {
int pivot = arr[high]; // Choose the rightmost element as pivot
int i = low - 1; // Index of smaller element
for (int j = low; j < high; j++) {
// If current element is smaller than or equal to pivot
if (arr[j] <= pivot) {
i++;
// Swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// Swap arr[i+1] and arr[high] (pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
// pi is partitioning index
int pi = partition(arr, low, high);
// Sort elements before and after partition
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
C- Time Complexity: O(n log n) average case, O(n²) worst case
Practice Exercise: Compare the performance of bubble sort and merge sort on arrays of different sizes.
Graph Algorithms
Breadth-First Search (BFS)
BFS explores all neighbors at the present depth before moving to nodes at the next depth level.
void BFS(std::vector<std::vector<int>>& graph, int start) {
int n = graph.size();
std::vector<bool> visited(n, false);
std::queue<int> queue;
visited[start] = true;
queue.push(start);
while (!queue.empty()) {
int current = queue.front();
queue.pop();
std::cout << current << " ";
for (int neighbor : graph[current]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
queue.push(neighbor);
}
}
}
}
C- Time Complexity: O(V + E) where V is vertices and E is edges
Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking.
void DFS(std::vector<std::vector<int>>& graph, int vertex, std::vector<bool>& visited) {
visited[vertex] = true;
std::cout << vertex << " ";
for (int neighbor : graph[vertex]) {
if (!visited[neighbor]) {
DFS(graph, neighbor, visited);
}
}
}
void DFSTraversal(std::vector<std::vector<int>>& graph, int start) {
int n = graph.size();
std::vector<bool> visited(n, false);
DFS(graph, start, visited);
}
C- Time Complexity: O(V + E) where V is vertices and E is edges
Practice Exercise: Implement a function to detect cycles in a directed graph using DFS.
Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems and storing the results to avoid redundant calculations.
Fibonacci Sequence
// Inefficient recursive approach
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n-1) + fibonacci(n-2);
}
// Efficient dynamic programming approach
int fibonacciDP(int n) {
if (n <= 1) return n;
int fib[n+1];
fib[0] = 0;
fib[1] = 1;
for (int i = 2; i <= n; i++) {
fib[i] = fib[i-1] + fib[i-2];
}
return fib[n];
}
CLongest Common Subsequence
int longestCommonSubsequence(std::string text1, std::string text2) {
int m = text1.length();
int n = text2.length();
std::vector<std::vector<int>> dp(m+1, std::vector<int>(n+1, 0));
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (text1[i-1] == text2[j-1]) {
dp[i][j] = dp[i-1][j-1] + 1;
} else {
dp[i][j] = std::max(dp[i-1][j], dp[i][j-1]);
}
}
}
return dp[m][n];
}
CPractice Exercise: Solve the “Coin Change” problem using dynamic programming: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
Problem-Solving Approaches
Divide and Conquer
This approach breaks a problem into smaller subproblems, solves them recursively, and combines their solutions.
Examples:
- Merge Sort
- Quick Sort
- Binary Search
Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step with the hope of finding a global optimum.
Examples:
- Dijkstra’s Shortest Path
- Huffman Coding
- Fractional Knapsack Problem
Backtracking
Backtracking builds candidates to a solution incrementally, abandoning a candidate as soon as it determines that the candidate cannot be completed to a valid solution.
Examples:
- N-Queens Problem
- Sudoku Solver
- Hamiltonian Path
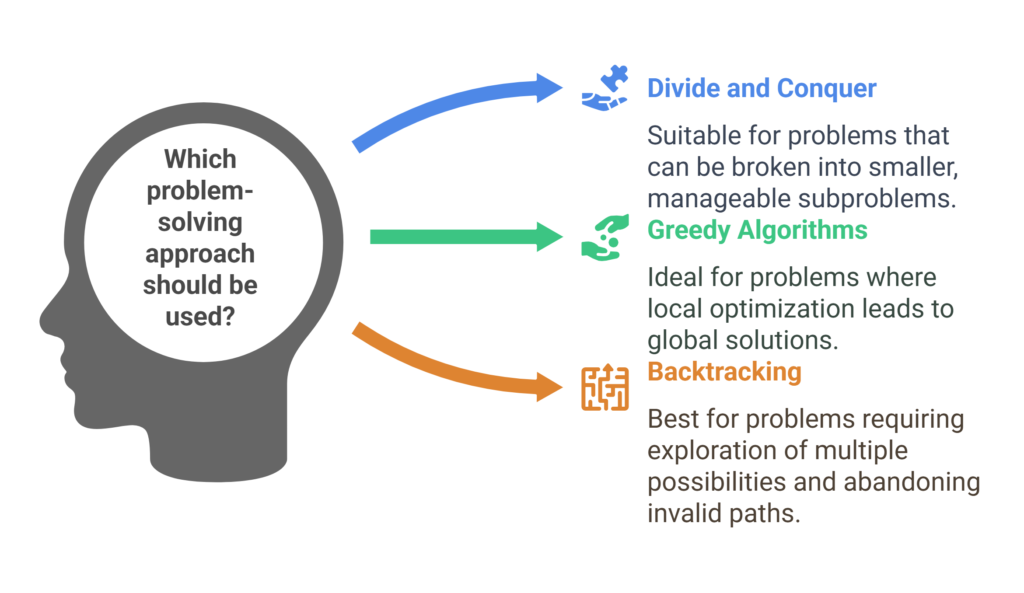
Practical Applications of DSA
Coding Interviews
Data structures and algorithms form the core of technical interviews at major tech companies. Understanding DSA is crucial for solving coding challenges efficiently and demonstrating your problem-solving skills.
Common Interview Topics:
- Array manipulation
- String processing
- Tree traversal
- Graph algorithms
- Dynamic programming
Real-World Applications
DSA knowledge translates directly to building better software:
- Web Development: Efficient data storage and retrieval in databases
- Mobile Apps: Optimizing performance on resource-constrained devices
- Game Development: Implementing complex game mechanics and AI
- Data Science: Processing and analyzing large datasets
- System Design: Building scalable and efficient systems
Best Practices for DSA
- Start with the basics: Master fundamental data structures before moving to complex ones
- Practice regularly: Solve problems on platforms like LeetCode, HackerRank, or CodeForces
- Analyze before coding: Understand the problem thoroughly before jumping into implementation
- Consider multiple approaches: There’s often more than one way to solve a problem
- Test edge cases: Consider boundary conditions and special inputs
- Optimize gradually: First make it work, then make it efficient
- Learn from others: Study efficient solutions and algorithms created by others
Common Mistakes to Avoid
- Premature optimization: Focusing too much on efficiency before ensuring correctness
- Using complex data structures unnecessarily: Sometimes simpler structures work better
- Ignoring space complexity: An algorithm might be time-efficient but memory-intensive
- Not understanding the problem domain: Choosing inappropriate data structures for the specific problem
- Reinventing the wheel: Not utilizing standard library implementations when available
FAQ: Common Questions About DSA
Do I need to learn all data structures and algorithms?
While it’s beneficial to have a broad understanding, focus on mastering the fundamental ones first. Then, expand your knowledge based on your specific interests or career goals.
Is DSA only important for coding interviews?
No, DSA knowledge is crucial for writing efficient code in any context, designing robust systems, and solving complex problems in software development.
Which programming language is best for learning DSA?
You can learn DSA in any language, but C++, Java, and Python are popular choices due to their widespread use and built-in data structure libraries.
How long does it take to master DSA?
Learning the basics might take a few weeks, but true mastery comes from continuous practice over months or years as you apply these concepts to increasingly complex problems.
Should I memorize algorithms?
Rather than memorizing, focus on understanding the underlying principles and problem-solving approaches. With practice, you’ll develop the ability to derive algorithms when needed.
How do I know which data structure to use for a problem?
Consider the operations you need to perform most frequently (insertion, deletion, search) and choose structures optimized for those operations. Experience with varied problems will improve your intuition.
Data Structures and Algorithms form the foundation of computer science and software development. By understanding how to store data efficiently and process it optimally, you’ll be equipped to solve complex problems, ace technical interviews, and build high-performance applications.
Remember that mastering DSA is a journey rather than a destination. Start with the fundamentals, practice regularly with diverse problems, and gradually tackle more complex concepts. The skills you develop will serve you throughout your programming career, regardless of the specific technologies or domains you work with.
As you continue your DSA journey, challenge yourself with increasingly difficult problems, collaborate with peers, and stay curious about new approaches and optimizations. With persistence and practice, you’ll develop the problem-solving mindset that distinguishes exceptional programmers.